SCALA
Scala Interview Questions
1) What is Scala?
Scala is a general-purpose programming language. It supports object oriented, functional and imperative programming approaches. It is a strong static type language. In Scala, everything is an object whether it is a function or a number.It was designed by Martin Odersky in 2004.
For more information:
2) What are the features of Scala?
There are following features in Scala:
- Type inference
- Singleton object
- Immutability
- Lazy computation
- Case classes and Pattern matching
- Concurrency control
- String interpolation
- Higher order function
- Traits
- Rich collection set and many more.
3) What are the Data Types in Scala?
Data types in Scala are much similar to java in terms of their storage, length, except that in Scala there is no concept of primitive data types every type is an object and starts with capital letter. A table of data types is given in the tutorials.
For more information: Click here
4) What is pattern matching?
Pattern matching is a feature of Scala. It works same as switch case in other languages. It matches best case available in the pattern.
For more information: Click here
5) What is for-comprehension in Scala?
In Scala, for loop is known as for-comprehensions. It can be used to iterate, filter and return an iterated collection. The for-comprehension looks a bit like a for-loop in imperative languages, except that it constructs a list of the results of all iterations.
For more information: Click here
6) What is breakable method in Scala?
In Scala, there is no break statement but you can do it by using break method and importing Scala.util.control.Breaks._ package. It can break your code.
For more information: Click here
7) How to declare function in Scala?
In Scala, functions are first class values. You can store function value, pass function as an argument and return function as a value from other function. You can create function by using def keyword. You must mention return type of parameters while defining function and return type of a function is optional. If you don't specify return type of a function, default return type is Unit.
For more information: Click here
8) Why do we use =(equal) operator in Scala function.
You can create function with or without = (equal) operator. If you use it, function will return value. If you don't use it, your function will not return anything and will work like subroutine.
For more information: Click here
9) What is Function parameter with default value in Scala?
Scala provides a feature to assign default values to function parameters. It helps in the scenario when you don't pass value during function calling. It uses default values of parameters.
For more information: Click here
10) What is function named parameter in Scala?
In Scala function, you can specify the names of parameters during calling the function. You can pass named parameters in any order and can also pass values only.
For more information: Click here
11) What is higher order function in Scala?
Higher order function is a function that either takes a function as argument or returns a function. In other words we can say a function which works with function is called higher order function.
For more information: Click here
12) What is function composition in Scala?
In Scala, functions can be composed from other functions. It is a process of composing in which a function represents the application of two composed functions.
For more information: Click here
13) What is Anonymous (lambda) Function in Scala?
Anonymous function is a function that has no name but works as a function. It is good to create an anonymous function when you don't want to reuse it latter.
You can create anonymous function either by using ⇒ (rocket) or _ (underscore) wild card in Scala.
For more information: Click here
14) What is multiline expression in Scala?
Expressions those are written in multiple lines are called multiline expression. In Scala, be carefull while using multiline expressions.
For more information: Click here
15) What is function currying in Scala?
In Scala, method may have multiple parameter lists. When a method is called with a fewer number of parameter lists, then this will yield a function taking the missing parameter lists as its arguments.
In other words it is a technique of transforming a function that takes multiple arguments into a function that takes a single argument.
For more information: Click here
16) What is nexted function in Scala?
In Scala, you can define function of variable length parameters. It allows you to pass any number of arguments at the time of calling the function.
For more information: Click here
17) What is object in Scala?
Object is a real world entity. It contains state and behavior. Laptop, car, cell phone are the real world objects. Object typically has two characteristics:
1) State: data values of an object are known as its state.
2) Behavior: functionality that an object performs is known as its behavior.
Object in Scala is an instance of class. It is also known as runtime entity.
For more information: Click here
18) What is class in Scala?
Class is a template or a blueprint. It is also known as collection of objects of similar type.
In Scala, a class can contain:
- Data member
- Member method
- Constructor
- Block
- Nested class
- Super class information etc.
For more information: Click here
19) What is anonymous object in Scala?
In Scala, you can create anonymous object. An object which has no reference name is called anonymous object. It is good to create anonymous object when you don't want to reuse it further.
For more information: Click here
20) What is constructor in Scala?
In Scala, constructor is not special method. Scala provides primary and any number of auxiliary constructors.
In Scala, if you don't specify primary constructor, compiler creates a default primary constructor. All the statements of class body treated as part of constructor. It is also known as default constructor.
For more information: Click here
21) What is method overloading in Scala?
Scala provides method overloading feature which allows us to define methods of same name but having different parameters or data types. It helps to optimize code. You can achieve method overloading either by using different parameter list or different types of parameters.
For more information: Click here
22) What is this in Scala?
In Scala, this is a keyword and used to refer current object. You can call instance variables, methods, constructors by using this keyword.
For more information: Click here
23) What is Inheritance?
Inheritance is an object oriented concept which is used to reusability of code. You can achieve inheritance by using extendskeyword. To achieve inheritance a class must extend to other class. A class which is extended called super or parent class. a class which extends class is called derived or base class.
For more information: Click here
24) What is method overriding in Scala?
When a subclass has the same name method as defined in the parent class, it is known as method overriding. When subclass wants to provide a specific implementation for the method defined in the parent class, it overrides method from parent class.
In Scala, you must use either override keyword or override annotation to override methods from parent class.
For more information: Click here
25) What is final in Scala?
Final keyword in Scala is used to prevent inheritance of super class members into derived class. You can declare final variable, method and class also.
For more information: Click here
26) What is final class in Scala?
In Scala, you can create final class by using final keyword. Final class can't be inherited. If you make a class final, it can't be extended further.
For more information: Click here
27) What is abstract class in Scala?
A class which is declared with abstract keyword is known as abstract class. An abstract class can have abstract methods and non-abstract methods as well. Abstract class is used to achieve abstraction.
For more information: Click here
28) What is Scala Trait?
A trait is like an interface with a partial implementation. In Scala, trait is a collection of abstract and non-abstract methods. You can create trait that can have all abstract methods or some abstract and some non-abstract methods.
For more information: Click here
29) What is trait mixins in Scala?
In Scala, trait mixins means you can extend any number of traits with a class or abstract class. You can extend only traits or combination of traits and class or traits and abstract class.
It is necessary to maintain order of mixins otherwise compiler throws an error.
For more information: Click here
30) What is access modifier in Scala?
Access modifier is used to define accessibility of data and our code to the outside world. You can apply accessibly to class, trait, data member, member method and constructor etc. Scala provides least accessibility to access to all. You can apply any access modifier to your code according to your requirement.
In Scala, there are only three types of access modifiers.
- No modifier
- Protected
- Private
For more information: Click here
31) What is array in Scala?
In Scala, array is a combination of mutable values. It is an index based data structure. It starts from 0 index to n-1 where n is length of array.
Scala arrays can be generic. It means, you can have an Array[T], where T is a type parameter or abstract type. Scala arrays are compatible with Scala sequences - you can pass an Array[T] where a Seq[T] is required. Scala arrays also support all the sequence operations.
For more information: Click here
32) What is ofDim method in Scala?
Scala provides an ofDim method to create multidimensional array. Multidimensional array is an array which store data in matrix form. You can create from two dimensional to three, four and many more dimensional array according to your need.
For more information: Click here
33) What is String in Scala?
In Scala, string is a combination of characters or we can say it is a sequence of characters. It is index based data structure and use linear approach to store data into memory. String is immutable in Scala like java.
For more information: Click here
34) What is string interpolation in Scala?
Starting in Scala 2.10.0, Scala offers a new mechanism to create strings from your data. It is called string interpolation. String interpolation allows users to embed variable references directly in processed string literals. Scala provides three string interpolation methods: s, f and raw.
For more information: Click here
35) What does s method in Scala String interpolation?
The s method of string interpolation allows us to pass variable in string object. You don't need to use + operator to format your output string. This variable is evaluated by compiler and variable is replaced by value.
For more information: Click here
36) What does f method in Scala String interpolation?
The f method is used to format your string output. It is like printf function of C language which is used to produce formatted output. You can pass your variables of any type in the print function.
For more information: Click here
37) What does raw method in Scala String interpolation?
The raw method of string interpolation is used to produce raw string. It does not interpret special char present in the string.
For more information: Click here
38) What is exception handling in Scala?
Exception handling is a mechanism which is used to handle abnormal conditions. You can also avoid termination of your program unexpectedly.
Scala makes "checked vs unchecked" very simple. It doesn't have checked exceptions. All exceptions are unchecked in Scala, even SQLException and IOException.
For more information: Click here
39) What is try catch in Scala?
Scala provides try and catch block to handle exception. The try block is used to enclose suspect code. The catch block is used to handle exception occurred in try block. You can have any number of try catch block in your program according to need.
For more information: Click here
40) What is finally in Scala?
The finally block is used to release resources during exception. Resources may be file, network connection, database connection etc. the finally block executes guaranteed.
41) What is throw in Scala?
You can throw exception explicitly in you code. Scala provides throw keyword to throw exception. The throw keyword mainly used to throw custom exception.
For more information: Click here
42) What is exception propagation in Scala?
In Scala, you can propagate exception in calling chain. When an exception occurs in any function it looks for handler. If handler not available there, it forwards to caller method and look for handler there. If handler present there, handler catch that exception. If handler not present it moves to next caller method in calling chain. This whole process is known as exception propagation.
43) What is throws in Scala?
Scala provides throws keyword to declare exception. You can declare exception with method definition. It provides information to the caller function that this method may throw this exception. It helps to caller function to handle and enclose that code in try-catch block to avoid abnormal termination of program. In Scala, you can either use throws keyword or throws annotation to declare exception.
For more information: Click here
44) What is custom exception in Scala?
In Scala, you can create your own exception. It is also known as custom exceptions. You must extend Exception class to while declaring custom exception class. You can create your own message in custom class.
For more information: Click here
45) What is collection in Scala?
Scala provides rich set of collection library. It contains classes and traits to collect data. These collections can be mutable or immutable. You can use them according to your requirement.
For more information: Click here
46) What is traversable in Scala collection?
It is a trait and used to traverse collection elements. It is a base trait for all Scala collections. It contains the methods which are common to all collections.
For more information: Click here
47) What does Set in Scala collection?
It is used to store unique elements in the set. It does not maintain any order for storing elements. You can apply various operations on them. It is defined in the Scala.collection.immutable package.
for more information: Click here
48) What does SortedSet in Scala collection?
In Scala, SortedSet extends Set trait and provides sorted set elements. It is useful when you want sorted elements in the Set collection. You can sort integer values and string as well.
It is a trait and you can apply all the methods defined in the traversable trait and Set trait.
for more information: Click here
49) What is HashSet in Scala collection?
HashSet is a sealed class. It extends AbstractSet and immutable Set trait. It uses hash code to store elements.
It neither maintains insertion order nor sorts the elements.
For more information: Click here
50) What is BitSet in Scala?
Bitsets are sets of non-negative integers which are represented as variable-size arrays of bits packed into 64-bit words. The memory footprint of a bitset is determined by the largest number stored in it. It extends Set trait.
For more information: Click here
51) What is ListSet in Scala collection?
In Scala, ListSet class implements immutable sets using a list-based data structure.
Elements are stored internally in reversed insertion order, which means the newest element is at the head of the list. It maintains insertion order.
This collection is suitable only for a small number of elements.
For more information: Click here
52) What is Seq in Scala collection?
Seq is a trait which represents indexed sequences that are guaranteed immutable. You can access elements by using their indexes. It maintains insertion order of elements.
Sequences support a number of methods to find occurrences of elements or subsequences.
It returns a list.
For more information: Click here
53) What is Vector in Scala collection?
Vector is a general-purpose, immutable data structure. It provides random access of elements. It is good for large collection of elements.
It extends an abstract class AbstractSeq and IndexedSeq trait.
For more information: Click here
54) What is List in Scala Collection?
List is used to store ordered elements. It extends LinearSeq trait. It is a class for immutable linked lists. This class is good for last-in-first-out (LIFO), stack-like access patterns.
It maintains order, can contain duplicates elements.
For more information: Click here
55) What is Queue in Scala Collection?
Queue implements a data structure that allows inserting and retrieving elements in a first-in-first-out (FIFO) manner.
In Scala, Queue is implemented as a pair of lists. One is used to insert the elements and second to contain deleted elements. Elements are added to the first list and removed from the second list.
For more information: Click here
56) What is stream in Scala?
Stream is a lazy list. It evaluates elements only when they are required. This is a feature of Scala. Scala supports lazy computation. It increases performance of your program.
For more information: Click here
57) What does Map in Scala Collection?
Map is used to store elements. It stores elements in pairs of key and values. In Scala, you can create map by using two ways either by using comma separated pairs or by using rocket operator.
For more information: Click here
58) What does ListMap in Scala?
This class implements immutable maps by using a list-based data structure. It maintains insertion order and returns ListMap. This collection is suitable for small elements.
You can create empty ListMap either by calling its constructor or using ListMap.empty method.
For more information: Click here
59) What is tuple in Scala?
A tuple is a collection of elements in ordered form. If there is no element present, it is called empty tuple. You can use tuple to store any type of data. You can store similar type to mix type data. You can return multiple values by using tuple in function.
For more information: Click here
60) What is singleton object in Scala?
Singleton object is an object which is declared by using object keyword instead by class. No object is required to call methods declared inside singleton object.
In Scala, there is no static concept. So Scala creates a singleton object to provide entry point for your program execution.
For more information: Click here
61) What is companion object in Scala?
In Scala, when you have a class with same name as singleton object, it is called companion class and the singleton object is called companion object.
The companion class and its companion object both must be defined in the same source file.
For more information: Click here
62) What are case classes in Scala?
Scala case classes are just regular classes which are immutable by default and decomposable through pattern matching.
It uses equal method to compare instance structurally.
It does not use new keyword to instantiate object.
For more information: Click here
63) What is file handling in Scala?
File handling is a mechanism of handling file operations. Scala provides predefined methods to deal with file. You can create, open, write and read file. Scala provides a complete package scala.io for file handling.
For more information: Click here
Here’s the list of questions I decided to answer to exercise myself, mostly from that repository:
- What is the difference between a
var
, a val
and def
?
- What is the difference between a
trait
and an abstract class
?
- What is the difference between an
object
and a class
?
- What is a
case class
?
- What is the difference between a Java future and a Scala future?
- What is the difference between
unapply
and apply
, when would you use them?
- What is a companion object?
- What is the difference between the following terms and types in Scala:
Nil
, Null
, None
, Nothing
?
- What is
Unit
?
- What is the difference between a
call-by-value
and call-by-name
parameter?
- Define uses for the
Option
monad and good practices it provides.
- How does
yield
work?
- Explain the implicit parameter precedence.
- What operations is a
for comprehension
syntactic sugar for?
- Streams:
- What consideration you need to have when you use Scala’s
Streams
?
- What technique does the Scala’s
Streams
use internally?
- What is a
value class
?
- Option vs Try vs Either
- What is function currying?
- What is Tail recursion?
- What are High Order Functions?
Here’s the list of questions I decided to answer to exercise myself, mostly from that repository:
- What is the difference between a
var
, aval
anddef
? - What is the difference between a
trait
and anabstract class
? - What is the difference between an
object
and aclass
? - What is a
case class
? - What is the difference between a Java future and a Scala future?
- What is the difference between
unapply
andapply
, when would you use them? - What is a companion object?
- What is the difference between the following terms and types in Scala:
Nil
,Null
,None
,Nothing
? - What is
Unit
? - What is the difference between a
call-by-value
andcall-by-name
parameter? - Define uses for the
Option
monad and good practices it provides. - How does
yield
work? - Explain the implicit parameter precedence.
- What operations is a
for comprehension
syntactic sugar for? - Streams:
- What consideration you need to have when you use Scala’s
Streams
? - What technique does the Scala’s
Streams
use internally?
- What consideration you need to have when you use Scala’s
- What is a
value class
? - Option vs Try vs Either
- What is function currying?
- What is Tail recursion?
- What are High Order Functions?
Answers
Before you decide to read my answers (which may even not be right - but I do hope they are
), try to answer them yourself.
Before you decide to read my answers (which may even not be right - but I do hope they are
), try to answer them yourself.

1. What is the difference between a var
, a val
and def
?
A var
is a variable. It’s a mutable reference to a value. Since it’s mutable, its value may change through the program lifetime. Keep in mind that the variable type cannot change in Scala. You may say that a var
behaves similarly to Java variables.
A val
is a value. It’s an immutable reference, meaning that its value never changes. Once assigned it will always keep the same value. It’s similar to constants in another languages.
A def
creates a method (and a method is different from a function - thanks to AP for his comment). It is evaluated on call.
var x = 3 // x is of type Int. If you force it to be of type Any then this example would work
x = 4 // accepted by the language/compiler
x = "error" // not accepted by the compiler
val y = 3
y = 4 // would produce an error 'error: reassignment to val'
def fun(name: String) = "Hey! My name is: " + name
fun("Scala") // "Hey! My name is: Scala"
fun("Java") // "Hey! My name is: Java"
Bonus: what’s a lazy val
? It’s almost like a val
, but its value is only computed when needed. It’s specially useful to avoid heavy computations (using short-circuit for instance).
lazy val x = {
println("computing x")
3
}
val y = {
println("computing y")
10
}
y + y // x was still not computed, "computing x" was not yet printed
x + x // x is required, x is going to be computed, a "computing x" message will be printed *once*
A
var
is a variable. It’s a mutable reference to a value. Since it’s mutable, its value may change through the program lifetime. Keep in mind that the variable type cannot change in Scala. You may say that a var
behaves similarly to Java variables.
A
val
is a value. It’s an immutable reference, meaning that its value never changes. Once assigned it will always keep the same value. It’s similar to constants in another languages.
A
def
creates a method (and a method is different from a function - thanks to AP for his comment). It is evaluated on call.var x = 3 // x is of type Int. If you force it to be of type Any then this example would work
x = 4 // accepted by the language/compiler
x = "error" // not accepted by the compiler
val y = 3
y = 4 // would produce an error 'error: reassignment to val'
def fun(name: String) = "Hey! My name is: " + name
fun("Scala") // "Hey! My name is: Scala"
fun("Java") // "Hey! My name is: Java"
Bonus: what’s a
lazy val
? It’s almost like a val
, but its value is only computed when needed. It’s specially useful to avoid heavy computations (using short-circuit for instance).lazy val x = {
println("computing x")
3
}
val y = {
println("computing y")
10
}
y + y // x was still not computed, "computing x" was not yet printed
x + x // x is required, x is going to be computed, a "computing x" message will be printed *once*
2. What is the difference between a trait
and an abstract class
?
The first difference is that a class
can only extend one other class
, but an unlimited number of traits
.
While traits
only support type parameters
, abstract classes
can have constructor parameters
.
Also, abstract classes
are interoperable with Java, while traits
are only interoperable with Java if they do not contain any implementation.
The first difference is that a
class
can only extend one other class
, but an unlimited number of traits
.
While
traits
only support type parameters
, abstract classes
can have constructor parameters
.
Also,
abstract classes
are interoperable with Java, while traits
are only interoperable with Java if they do not contain any implementation.
3. What is the difference between an object
and a class
?
An object
is a singleton instance of a class
. It does not need to be instantiated by the developer.
If an object
has the same name that a class
, the object
is called a companion object
(check Q7 for more details).
class MyClass(number: Int, text: String) {
def classMethod() = ???
}
object MyObject {
def objectMethod() = ???
}
new MyClass(3, "text").classMethod()
MyClass.classMethod() // won't compile
MyObject.objectMethod() // you don't need to create an instance to call the method
An
object
is a singleton instance of a class
. It does not need to be instantiated by the developer.
If an
object
has the same name that a class
, the object
is called a companion object
(check Q7 for more details).class MyClass(number: Int, text: String) {
def classMethod() = ???
}
object MyObject {
def objectMethod() = ???
}
new MyClass(3, "text").classMethod()
MyClass.classMethod() // won't compile
MyObject.objectMethod() // you don't need to create an instance to call the method
4. What is a case class
?
A case class
is syntactic sugar for a class that is immutable and decomposable through pattern matching (because they have an apply
and unapply
methods). Being decomposable means it is possible to extract its constructors parameters in the pattern matching.
Case classes contain a companion object which holds the apply
method. This fact makes possible to instantiate a case class without the new
keyword. They also come with some helper methods like the .copy
method, that eases the creation of a slightly changed copy from the original.
Finally, case classes are compared by structural equality instead of being compared by reference, i.e., they come with a method which compares two case classes by their values/fields, instead of comparing just the references.
Case classes are specially useful to be used as DTOs.
case class MyCaseClass(number: Int, text: String, others: List[Int])
val dto = MyCaseClass(3, "text", List.empty)
dto.copy(number = 5) // will produce an instance equal to the original, with number = 5 instead of 3
val dto2 = MyCaseClass(3, "text", List.empty)
dto == dto2 // will return true even if different references
class MyClass(number: Int, text: String, others: List[Int]) {}
val c1 = new MyClass(1, "txt", List.empty)
val c2 = new MyClass(1, "txt", List.empty)
c1 == c2 // will return false because they are different references
A
case class
is syntactic sugar for a class that is immutable and decomposable through pattern matching (because they have an apply
and unapply
methods). Being decomposable means it is possible to extract its constructors parameters in the pattern matching.
Case classes contain a companion object which holds the
apply
method. This fact makes possible to instantiate a case class without the new
keyword. They also come with some helper methods like the .copy
method, that eases the creation of a slightly changed copy from the original.
Finally, case classes are compared by structural equality instead of being compared by reference, i.e., they come with a method which compares two case classes by their values/fields, instead of comparing just the references.
Case classes are specially useful to be used as DTOs.
case class MyCaseClass(number: Int, text: String, others: List[Int])
val dto = MyCaseClass(3, "text", List.empty)
dto.copy(number = 5) // will produce an instance equal to the original, with number = 5 instead of 3
val dto2 = MyCaseClass(3, "text", List.empty)
dto == dto2 // will return true even if different references
class MyClass(number: Int, text: String, others: List[Int]) {}
val c1 = new MyClass(1, "txt", List.empty)
val c2 = new MyClass(1, "txt", List.empty)
c1 == c2 // will return false because they are different references
5. What is the difference between a Java future and a Scala future?
This one I had to google a little about it. I have never used Java futures, so it was impossible for me to answer.
Obviously, I was not the first to search for the differences between both futures. I found a really clean and simple answer on StackOverflow which highlights the fact that the Scala implementation is in fact asynchronous without blocking, while in Java you can’t get the future value without blocking.
Scala provides an API to manipulate the future as a monad or by attaching callbacks for completion. Unless you decide to use the Await
, you won’t block your program using Scala futures.
This one I had to google a little about it. I have never used Java futures, so it was impossible for me to answer.
Obviously, I was not the first to search for the differences between both futures. I found a really clean and simple answer on StackOverflow which highlights the fact that the Scala implementation is in fact asynchronous without blocking, while in Java you can’t get the future value without blocking.
Scala provides an API to manipulate the future as a monad or by attaching callbacks for completion. Unless you decide to use the
Await
, you won’t block your program using Scala futures.
6. What is the difference between unapply
and apply
, when would you use them?
unapply
is a method that needs to be implemented by an object in order for it to be an extractor. Extractors are used in pattern matching to access an object constructor parameters. It’s the opposite of a constructor.
The apply
method is a special method that allows you to write someObject(params)
instead of someObject.apply(params)
. This usage is common in case classes
, which contain a companion object with the apply
method that allows the nice syntax to instantiate a new object without the new
keyword.
unapply
is a method that needs to be implemented by an object in order for it to be an extractor. Extractors are used in pattern matching to access an object constructor parameters. It’s the opposite of a constructor.
The
apply
method is a special method that allows you to write someObject(params)
instead of someObject.apply(params)
. This usage is common in case classes
, which contain a companion object with the apply
method that allows the nice syntax to instantiate a new object without the new
keyword.7. What is a companion object?
If an object
has the same name that a class
, the object is called a companion object
. A companion object has access to methods of private visibility of the class, and the class also has access to private methods of the object. Doing the comparison with Java, companion objects hold the “static methods” of a class.
Note that the companion object has to be defined in the same source file that the class.
class MyClass(number: Int, text: String) {
private val classSecret = 42
def x = MyClass.objectSecret + "?" // MyClass.objectSecret is accessible because it's inside the class. External classes/objects can't access it
}
object MyClass { // it's a companion object because it has the same name
private val objectSecret = "42"
def y(arg: MyClass) = arg.classSecret -1 // arg.classSecret is accessible because it's inside the companion object
}
MyClass.objectSecret // won't compile
MyClass.classSecret // won't compile
new MyClass(-1, "random").objectSecret // won't compile
new MyClass(-1, "random").classSecret // won't compile
If an
object
has the same name that a class
, the object is called a companion object
. A companion object has access to methods of private visibility of the class, and the class also has access to private methods of the object. Doing the comparison with Java, companion objects hold the “static methods” of a class.
Note that the companion object has to be defined in the same source file that the class.
class MyClass(number: Int, text: String) {
private val classSecret = 42
def x = MyClass.objectSecret + "?" // MyClass.objectSecret is accessible because it's inside the class. External classes/objects can't access it
}
object MyClass { // it's a companion object because it has the same name
private val objectSecret = "42"
def y(arg: MyClass) = arg.classSecret -1 // arg.classSecret is accessible because it's inside the companion object
}
MyClass.objectSecret // won't compile
MyClass.classSecret // won't compile
new MyClass(-1, "random").objectSecret // won't compile
new MyClass(-1, "random").classSecret // won't compile
8. What is the difference between the following terms and types in Scala: Nil
, Null
, None
, Nothing
?
The None
is the empty representation of the Option
monad (see answer #11).
Null
is a Scala trait, where null
is its only instance. The null
value comes from Java and it’s an instance of any object, i.e., it is a subtype of all reference types, but not of value types. It exists so that reference types can be assigned null
and value types (like Int
or Long
) can’t.
Nothing
is another Scala trait
. It’s a subtype of any other type, and it has no subtypes. It exists due to the complex type system Scala has. It has zero instances. It’s the return type of a method that never returns normally, for instance, a method that always throws an exception. The reason Scala has a bottom type is tied to its ability to express variance in type parameters..
Finally, Nil
represents an empty List of anything of size zero. Nil
is of type List[Nothing]
.
All these types can create a sense of emptiness right? Here’s a little help understanding emptiness in Scala.
The
None
is the empty representation of the Option
monad (see answer #11).Null
is a Scala trait, where null
is its only instance. The null
value comes from Java and it’s an instance of any object, i.e., it is a subtype of all reference types, but not of value types. It exists so that reference types can be assigned null
and value types (like Int
or Long
) can’t.Nothing
is another Scala trait
. It’s a subtype of any other type, and it has no subtypes. It exists due to the complex type system Scala has. It has zero instances. It’s the return type of a method that never returns normally, for instance, a method that always throws an exception. The reason Scala has a bottom type is tied to its ability to express variance in type parameters..
Finally,
Nil
represents an empty List of anything of size zero. Nil
is of type List[Nothing]
.
All these types can create a sense of emptiness right? Here’s a little help understanding emptiness in Scala.
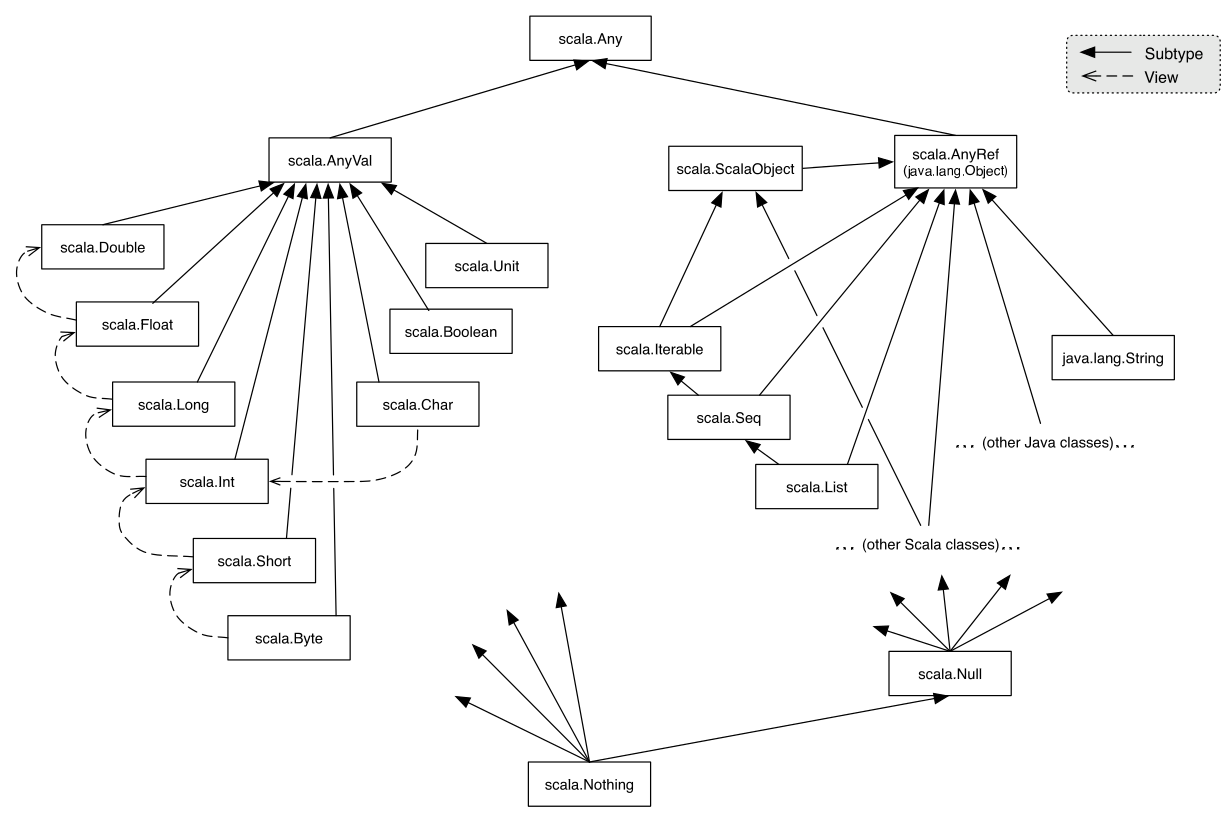
9. What is Unit
?
Unit
is a type which represents the absence of value, just like Java void
. It is a subtype of scala.AnyVal
. There is only one value of type Unit, represented by ()
, and it is not represented by any object in the underlying runtime system.
Unit
is a type which represents the absence of value, just like Java void
. It is a subtype of scala.AnyVal
. There is only one value of type Unit, represented by ()
, and it is not represented by any object in the underlying runtime system.
10. What is the difference between a call-by-value
and call-by-name
parameter?
The difference between a call-by-value
and a call-by-name
parameter, is that the former is computed before calling the function, and the later is evaluated when accessed.
Example: If we declare the following functions
def func(): Int = {
println("computing stuff....")
42 // return something
}
def callByValue(x: Int) = {
println("1st x: " + x)
println("2nd x: " + x)
}
def callByName(x: => Int) = {
println("1st x: " + x)
println("2nd x: " + x)
}
and now call them:
scala> callByValue(func())
computing stuff....
1st x: 42
2nd x: 42
scala> callByName(func())
computing stuff....
1st x: 42
computing stuff....
2nd x: 42
As it may be seen, the call-by-name
example makes the computation only when needed, and every time it is called, while the call-by-value
only computes once, but it computes before invoking the function (callByName
).
The difference between a
call-by-value
and a call-by-name
parameter, is that the former is computed before calling the function, and the later is evaluated when accessed.
Example: If we declare the following functions
def func(): Int = {
println("computing stuff....")
42 // return something
}
def callByValue(x: Int) = {
println("1st x: " + x)
println("2nd x: " + x)
}
def callByName(x: => Int) = {
println("1st x: " + x)
println("2nd x: " + x)
}
and now call them:
scala> callByValue(func())
computing stuff....
1st x: 42
2nd x: 42
scala> callByName(func())
computing stuff....
1st x: 42
computing stuff....
2nd x: 42
As it may be seen, the
call-by-name
example makes the computation only when needed, and every time it is called, while the call-by-value
only computes once, but it computes before invoking the function (callByName
).
11. Define uses for the Option
monad and good practices it provides.
The Option
monad is the Scala solution to the null
problem from Java. While in Java the absence of a value is modeled through the null
value, in Scala its usage is discouraged, in flavor of the Option
.
Using null
values one might try to call a method on a null instance, because the developer was not expecting that there could be no value, and get a NullPointerException
. Using the Option
, the developer always knows in which cases it may have to deal with the absence of value.
val person: Person = getPersonByIdOnDatabaseUnsafe(id = 4) // returns null if no person for provided id
println(s"This person age is ${person.age}") // if null it will throw an exception
val personOpt: Option[Person] = getPersonByIdOnDatabaseSafe(id = 4) // returns an empty Option if no person for provided id
personOpt match {
case Some(p) => println(s"This person age is ${p.age}")
case None => println("There is no person with that id")
}
The
Option
monad is the Scala solution to the null
problem from Java. While in Java the absence of a value is modeled through the null
value, in Scala its usage is discouraged, in flavor of the Option
.
Using
null
values one might try to call a method on a null instance, because the developer was not expecting that there could be no value, and get a NullPointerException
. Using the Option
, the developer always knows in which cases it may have to deal with the absence of value.val person: Person = getPersonByIdOnDatabaseUnsafe(id = 4) // returns null if no person for provided id
println(s"This person age is ${person.age}") // if null it will throw an exception
val personOpt: Option[Person] = getPersonByIdOnDatabaseSafe(id = 4) // returns an empty Option if no person for provided id
personOpt match {
case Some(p) => println(s"This person age is ${p.age}")
case None => println("There is no person with that id")
}
12. How does yield
work?
yield
generates a value to be kept in each iteration of a loop. yield
is used in for comprehensions as to provide a syntactic alternative to the combined usage of map
/flatMap
and filter
operations on monads (see answer #14).
scala> for (i <- 1 to 5) yield i * 2
res0: scala.collection.immutable.IndexedSeq[Int] = Vector(2, 4, 6, 8, 10)
yield
generates a value to be kept in each iteration of a loop. yield
is used in for comprehensions as to provide a syntactic alternative to the combined usage of map
/flatMap
and filter
operations on monads (see answer #14).scala> for (i <- 1 to 5) yield i * 2
res0: scala.collection.immutable.IndexedSeq[Int] = Vector(2, 4, 6, 8, 10)
13. Explain the implicit parameter precedence.
Implicit parameters can lead to unexpected behavior if one is not aware of the precedence when looking up.
So, what’s the order the compiler will look up for implicits?
- implicits declared locally
- imported implicits
- outer scope (implicits declared in the class are considered outer scope in a class method for instance)
- inheritance
- package object
- implicit scope like companion objects
Implicit parameters can lead to unexpected behavior if one is not aware of the precedence when looking up.
So, what’s the order the compiler will look up for implicits?
- implicits declared locally
- imported implicits
- outer scope (implicits declared in the class are considered outer scope in a class method for instance)
- inheritance
- package object
- implicit scope like companion objects
14. What operations is a for comprehension
syntactic sugar for?
A for comprehension
is a alternative syntax for the composition of several operations on monads.
A for comprehension
can be replaced by foreach
operations (if no yield
keyword is being used), or by map
/flatMap
and filter
(actually, while confirming my words I found out about the withFilter
method).
for {
x <- c1
y <- c2
z <- c3 if z > 0
} yield {...}
is translated into
c1.flatMap(x => c2.flatMap(y => c3.withFilter(z => z > 0).map(z => {...})))
A
for comprehension
is a alternative syntax for the composition of several operations on monads.
A
for comprehension
can be replaced by foreach
operations (if no yield
keyword is being used), or by map
/flatMap
and filter
(actually, while confirming my words I found out about the withFilter
method).for {
x <- c1
y <- c2
z <- c3 if z > 0
} yield {...}
is translated into
c1.flatMap(x => c2.flatMap(y => c3.withFilter(z => z > 0).map(z => {...})))
15. Streams: What consideration you need to have when you use Scala’s Streams
? What technique does the Scala’s Streams
use internally?
While Scala Streams
can be really useful due to its lazy nature, it may also come with some unexpected problems.
The biggest problem is that Scala Streams
can be infinite, but your memory isn’t. If used wrongly, streams can lead to memory consumption problems. One must be cautious when saving references to a stream
. One common guideline, is not to assign a stream (head) to a val
, but instead, make it a def
.
This is a consequence of the technique behind streams
: memoization
While Scala
Streams
can be really useful due to its lazy nature, it may also come with some unexpected problems.
The biggest problem is that Scala
Streams
can be infinite, but your memory isn’t. If used wrongly, streams can lead to memory consumption problems. One must be cautious when saving references to a stream
. One common guideline, is not to assign a stream (head) to a val
, but instead, make it a def
.
This is a consequence of the technique behind
streams
: memoization
16. What is a value class
?
Have you ever had one of those nasty bugs where you were using an integer
thinking it would represent something but it actually represented a totally different thing ? For instance, an integer
representing an age, and another representing an height getting mixed (180 years old and 25 centimetres tall do look weird no?).
Because of that, it’s considered a good practice to wrap primitive types into more meaningful types.
Value classes allow a developer to increase the program type safety without incurring into penalties from allocating runtime objects.
There are some constraints and limitations, but the basic idea is that at compile time the object allocation is removed, by replacing the value classes instance by primitive types. More details can be found on its SIP.
Have you ever had one of those nasty bugs where you were using an
integer
thinking it would represent something but it actually represented a totally different thing ? For instance, an integer
representing an age, and another representing an height getting mixed (180 years old and 25 centimetres tall do look weird no?).
Because of that, it’s considered a good practice to wrap primitive types into more meaningful types.
Value classes allow a developer to increase the program type safety without incurring into penalties from allocating runtime objects.
There are some constraints and limitations, but the basic idea is that at compile time the object allocation is removed, by replacing the value classes instance by primitive types. More details can be found on its SIP.
17. Option vs Try vs Either
All of these 3 monads allow us to represent a computation that did not executed as expected.
An Option
, as explained on answer #11, represents the absence of value. It can be used when searching for something. For instance, database accesses often return Option
in lookup queries.
Try
is the monad approach to the Java try/catch
block. It wraps runtime exceptions.
If you need to provide a little more info about the reason the computation has failed, Either
may be very useful. With Either
you specify two possible return types: the expected/correct/successful and the error case which can be as simple as a String
message to be displayed to the user, or a full ADT.
def personAge(id: Int): Either[String, Int] = {
val personOpt: Option[Person] = DB.getPersonById(id)
personOpt match {
case None => Left(s"Could not get person with id: $id")
case Some(person) => Right(person.age)
}
}
All of these 3 monads allow us to represent a computation that did not executed as expected.
An
Option
, as explained on answer #11, represents the absence of value. It can be used when searching for something. For instance, database accesses often return Option
in lookup queries.Try
is the monad approach to the Java try/catch
block. It wraps runtime exceptions.
If you need to provide a little more info about the reason the computation has failed,
Either
may be very useful. With Either
you specify two possible return types: the expected/correct/successful and the error case which can be as simple as a String
message to be displayed to the user, or a full ADT.def personAge(id: Int): Either[String, Int] = {
val personOpt: Option[Person] = DB.getPersonById(id)
personOpt match {
case None => Left(s"Could not get person with id: $id")
case Some(person) => Right(person.age)
}
}
18. What is function currying?
Currying is a technique of making a function that takes multiple arguments into a series of functions that take a part of the arguments.
def add(a: Int)(b: Int) = a + b
val add2 = add(2)(_)
scala> add2(3)
res0: Int = 5
Currying is useful in many different contexts, but most often when you have to deal with Higher order functions
.
Currying is a technique of making a function that takes multiple arguments into a series of functions that take a part of the arguments.
def add(a: Int)(b: Int) = a + b
val add2 = add(2)(_)
scala> add2(3)
res0: Int = 5
Currying is useful in many different contexts, but most often when you have to deal with
Higher order functions
.19. What is Tail recursion?
In “normal” recursive methods, a method calls itself at some point. This technique is used in the naive implementation of the Fibonacci number, for instance. The problem with this approach is that at each recursive step, another chunk of information needs to be saved on the stack. In some cases, an huge number of recursive steps can occur, leading to stack overflow errors.
Tail recursion solves this problem. In tail recursive methods, all the computations are done before the recursive call, and the last statement is the recursive call. Compilers can then take advantage of this property to avoid stack overflow errors, since tail recursive calls can be optimized by not inserting info into the stack.
You can ask the compiler to enforce tail recursion in a method with @tailrec
def sum(n: Int): Int = { // computes the sum of the first n natural numbers
if(n == 0) {
n
} else {
n + sum(n - 1)
}
}
@tailrec // just to ensure at compile time
def tailSum(n: Int, acc: Int = 0): Int = {
if(n == 0) {
acc
} else {
tailSum(n - 1, acc + n)
}
}
if we now run both versions, what would happen?
> sum(5)
sum(5)
5 + sum(4) // computation on hold => needs to add info into the stack
5 + (4 + sum(3))
5 + (4 + (3 + sum(2)))
5 + (4 + (3 + (2 + sum(1))))
5 + (4 + (3 + (2 + 1)))
15
tailSum(5) // tailSum(5, 0) because the default value
tailSum(4, 5) // no computations on hold
tailSum(3, 9)
tailSum(2, 12)
tailSum(1, 14)
tailSum(0, 15)
15
In “normal” recursive methods, a method calls itself at some point. This technique is used in the naive implementation of the Fibonacci number, for instance. The problem with this approach is that at each recursive step, another chunk of information needs to be saved on the stack. In some cases, an huge number of recursive steps can occur, leading to stack overflow errors.
Tail recursion solves this problem. In tail recursive methods, all the computations are done before the recursive call, and the last statement is the recursive call. Compilers can then take advantage of this property to avoid stack overflow errors, since tail recursive calls can be optimized by not inserting info into the stack.
You can ask the compiler to enforce tail recursion in a method with @tailrec
def sum(n: Int): Int = { // computes the sum of the first n natural numbers
if(n == 0) {
n
} else {
n + sum(n - 1)
}
}
@tailrec // just to ensure at compile time
def tailSum(n: Int, acc: Int = 0): Int = {
if(n == 0) {
acc
} else {
tailSum(n - 1, acc + n)
}
}
if we now run both versions, what would happen?
> sum(5)
sum(5)
5 + sum(4) // computation on hold => needs to add info into the stack
5 + (4 + sum(3))
5 + (4 + (3 + sum(2)))
5 + (4 + (3 + (2 + sum(1))))
5 + (4 + (3 + (2 + 1)))
15
tailSum(5) // tailSum(5, 0) because the default value
tailSum(4, 5) // no computations on hold
tailSum(3, 9)
tailSum(2, 12)
tailSum(1, 14)
tailSum(0, 15)
15
20. What are High Order Functions?
High order functions are functions that can receive or return other functions. Common examples in Scala are the .filter
, .map
, .flatMap
functions, which receive other functions as arguments.
High order functions are functions that can receive or return other functions. Common examples in Scala are the
.filter
, .map
, .flatMap
functions, which receive other functions as arguments.
------------
Here is my list of some 20 odd questions from Scala programming and language in general. These are collected from various sources including friends and colleagues. It is mostly for Java developers who are learning Scala or have some experience working in Scala, looking for development job in Scala. Since the demand of Scala developer is on the rise, with preference to experienced Java programmers, you could use this list to prepare for telephonic and screening round of interviews.
What is the latest version of Scala?
This is a simple question to check whether you keep yourself up-to-date with the latest information about Scala or not. If you have not been using Scala actively and don't know the answer then you can say that you have used certain Scala version. Though, the current version of Scala is Scala 2.12, which also require Java 8. Older Scala versions are compatible with Java 6 and above.
Who is the author of Scala Programming language?
This is another basic question about Scala to check your general knowledge about Scala. It is designed by Martin Oderskey, a German computer scientist. He also teaches a course, Functional Programming Principles in Scala and Functional Program Design in Scala on Coursera.
What is the difference between val and var in Scala?
The val keyword stands for value and var stands for variable. You can use keyword val to store values, these are immutable, and cannot change once assigned. On the other hand, keyword var is used to create variables, which are values that can change after being set. If you try to modify a val, the compiler will throw an error. It is similar to the final variable in Java or const in C++.
What is the difference between == in Java and Scala?
Scala has more intuitive notion of equality. The == operator will automatically run the instance's equals method, rather than doing Java style comparison to check that two objects are actually the same reference. By the way, you can still check for referential equality by using eq method. In short, Java == operator compare references while Scala calls the equals() method. You can also read the difference between == and equals() in Java to learn more about how they behave in Java, here.
How to you create Singleton classes in Scala?
Scala introduces a new object keyword, which is used to represent Singleton classes. These are the class with just one instance and their method can be thought of as similar to Java's static methods. Here is a Singleton class in Scala:
package test
object Singleton{
def sum(l: List[Int]): Int = l.sum
}
This sum method is available globally, and can be referred to, or imported, as the test.Singleton.sum. A singleton object in Scala can also extend classes and traits.
Which books you have read on Scala so far?
This is a general question to check how did you learn Scala, did you read book or documentation or tutorial etc. It's best to name the book you have read on Scala e.g. I have read Programming in Scala by Martin Odersky and Functional Programming in Scala 1st Edition by Paul Chiusano. If you have not read any book then just say you have not read any book yet and like to read Scala documentation or planning to read a book etc. The most important thing is, to be honest, don't name a book if you have not read it yet. On a different note, try reading a good book on Scala, Martin Odersky's Programming in Scala: Updated for Scala 2.12 is a good starting point.
Which keyword is used to define a function in Scala?
A function is defined in Scala using the def keyword. This may sound familiar to Python developers as Python also uses def to define a function.
What is the use of App class in Scala?
Scala provides a helper class, called App, that provides the main method. Instead of writing your own main method, classes can extend App class to produce concise and executable applications in Scala as shown in the following example:
object Main extends App {
Console.println("Hello Scala: " + (args aString ", "))
}
Here, object Main inherits the main method of App trait and args returns the current command line arguments as an array, similar to String[] args in Java main method.
What's the difference between the following terms and types in Scala: 'Nil', 'Null', 'None', and 'Nothing' in Scala?
Answer: Even though they look similar, there are some subtle differences between them, let's see them one by one:
- Nil represents the end of a List.
- Null denotes the absence of value but in Scala, more precisely, Null is a type that represents the absence of type information for complex types that are inherited from AnyRef. It is different than null in Java.
- None is the value of an Option if it has no value in it.
- Nothing is the bottom type of the entire Scala type system, incorporating all types under AnyVal and AnyRef. Nothing is commonly used as a return type from a method that does not terminate normally and throws an exception
What is the difference between an object and a class in Scala?
An object is a singleton instance of a class. It does not need to be instantiated by the developer. If an object has the same name that a class, the object is called a companion object.
What is the difference between a trait and an abstract class in Scala?
Here are some key differences between a trait and an abstract class in Scala:
- A class can inherit from multiple traits but only one abstract class.
- Abstract classes can have constructor parameters as well as type parameters. Traits can have only type parameters. For example, you can’t say trait t(i: Int) {}; the i parameter is illegal.
- Abstract classes are fully interoperable with Java. You can call them from Java code without any wrappers. On the other hand, Traits are fully interoperable only if they do not contain any implementation code. See here to learn more about Abstract class in Java and OOP.
What is the difference between a call-by-value and call-by-name parameter?
The main difference between a call-by-value and a call-by-name parameter is that the former is computed before calling the function, and the later is evaluated when accessed.
What is the case class in Scala?
A case class is a special class definition, which works just like a regular Scala class but compiler creates equals() and toString() methods, as well as immutable access to the field of the class. You can construct case class instance without using the new keyword. One more benefit of the case classes is that instances can be decomposed into their constructed fields and can be used in pattern matching. Here is an example of case class hierarchy in Scala, which consists of an abstract super class Vehicle and two concrete case classes Car and Bike
abstract class Vehicle
case class Car(brand: String) extends Vehicle
case class Bike(brand: String, price: long) extends Vehicle
Instantiating a case class is easy because you don’t need to use the new keyword
val myCar = Car("BMW")
The constructor parameters of case classes are treated as public values and can be accessed directly e.g. myCar.brand will give you "BMW".
Here is another example of traits in Scala using case classes:
What is the difference between a Java method and a Scala function?
Scala function can be treated as a value. It can be assigned to a val or var, or even returned from another function, which is not possible in Java. Though Java 8 brings lambda expression which also makes function as a first class object, which means you can pass a function to a method just like you pass an object as an argument. See here to learn more about the difference between Scala and Java.
What is the difference between a Java future and a Scala future?
Even though both Java and Scala's Future object provides asynchronous computation, but there is a subtle difference between them on how you retrieve the result of the computation. Java's Future (java.util.concurrent.Future) requires that you access the result via a blocking get method.Although you can call isDone() method to find out if a Java Future has completed before calling get, thereby avoiding any blocking, you must wait until the Java Future has completed before proceeding with any computation that uses the result. With scala.concurrent.Future you can attach callbacks for completion (success/failure) or simply map it and chain multiple Futures together in a monadic fashion without blocking.
What is Companion Object in Scala?
If an object has the same name that a class, the object is called a companion object. A companion object has access to methods of private visibility of the class, and the class also has access to private methods of the object. Doing the comparison with Java, companion objects hold the "static methods" of a class. Just remember that the companion object has to be defined in the same source file that the class. You should also know that Scala classes cannot have static variables or methods. We commonly use Companion Objects for Factories.
What is tail recursion in Scala? What is the benefit?
A function is called tail recursive if the recursive call is the last operation in the function; the method does not rely on the result of the recursive call for any more computation. The most important benefit of the tail recursive function is that compiler can rewrite the method in an iterative fashion, similar to a while loop with mutable state. This allows long, deep recursion without taking too much of stack memory that could result in StackOverFlowError. Unfortunately, Java compiler doesn't support tail call optimization but Scala compiler does. In Scala, you can annotate a function with @tailrec to inform the compiler that this particular method is tail recursive. If the compiler cannot make the function tail recursive, it will throw an error.
What is "traits" in Scala?
Traits are basically Scala's workaround for the JVM not supporting multiple class inheritance. Here is an example of traits in Scala:
trait Car {
val brand: String
}
trait Costly{
val cost: Int
}
trait Milege{
val milege: Int
}
class Audi extends Car with Shiny with Miles{
val brand = "Audi"
val cost = 140000
val milege = 20
}
What is Akka, Play, and Sleek in Scala?
Akka is a concurrency framework in Scala which uses Actor based model for building highly concurrent, distributed, and resilient message-driven applications on the JVM. It uses high-level abstractions like Actor, Future, and Stream to simplify coding for concurrent applications. It also provides load balancing, routing, partitioning, and adaptive cluster management. If you are interested in learning Akka, I suggest reading Akka in Action and Akka documentation, both are great resources to learn Akka.
Play is another Scala framework to build web applications in both Java and Scala. The PlayFramework is based on a lightweight, stateless, web-friendly architecture. It is built on Akka, Play provides predictable and minimal resource consumption (CPU, memory, threads) for highly-scalable applications.
Slick is a modern database query and access library for Scala. It allows you to work with stored data almost as if you were using Scala collections while at the same time giving you full control over when a database access happens and which data is transferred. You can write your database queries in Scala instead of SQL, thus profiting from the static checking, compile-time safety, and compositionality of Scala. Slick features an extensible query compiler which can generate code for different backends.
What is Spark?
Apache Spark is the big data and cloud computing framework written in Scala. The Spark has been engineered from the bottom-up for performance, Spark can be 100x faster than Hadoop for large scale data processing by exploiting in memory computing and other optimizations. Spark is also fast when data is stored on disk, and currently, holds the world record for large-scale on-disk sorting. Many developers are now learning Scala for Spark to get into lucrative Big Data Job Market. I recommend reading "Advanced Analytics with Spark: Patterns for Learning from Data at Scale" by Sandy Ryza, Uri Laserson, Sean Owen, and Josh Wills. This book will also teach you how you can use functional programming techniques to solve real-world problems, which is a great skill to have.
What is 'Option' and how is it used in Scala?
The 'Option' in Scala is similar to Optional of Java 8. It is a wrapper type that avoids the occurrence of a NullPointerException in your code by giving you default value in case object is null. When you call get() from Option it can return a default value if the value is null. More importantly, Option provides the ability to differentiate within the type system those values that can be nulled and those that cannot be nulled.
What is ‘Unit’ and ‘()’ in Scala?
The 'Unit' is a type similar to void in Java. You can say it is a Scala equivalent of the void in Java, while still providing the language with an abstraction over the Java platform. The empty tuple '()' is a term representing a Unit value in Scala.
How to compile and run a Scala program?
You can use Scala compiler scalac to compile Scala program (similar to javac) and scala command to run them (similar to scala)
How to tell Scala to look into a class file for some Java class?
Similar to Java, you can use -classpath argument to include a JAR in Scala's classpath, as shown below
$ scala -classpath jar
Alternatively, you can also use CLASSPATH environment variable.
What is the difference between a normal class and a case class in Scala?
Following are some key differences between a case class and a normal class in Scala:
- case class allows pattern matching on it.
- you can create instances of case class without using the new keyword
- equals(), hashcode() and toString() method are automatically generated for case classes in Scala
- Scala automatically generate accessor methods for all constructor argument
What is the difference in between Scala and Java package?
Unlike Java, Scala also supports relative package names.
What is the difference between 'concurrency' and 'parallelism'? Name some constructs you can use in Scala to leverage both.
Concurrency is when several computations are executing sequentially during overlapping time periods, while parallelism describes processes that are executed simultaneously. The concepts are often confused because actors can be concurrent and parallel, sometimes simultaneously. For example, Node.js has concurrency via its event loop despite being a single threaded implementation. Parallel collections are a canonical example of parallelism, but Futures and the Async library can be as well. In short, Concurrency is about avoiding access to mutable state by multiple threads at the same time. Parallelism is taking a single task and breaking it apart to be performed by multiple threads at the same time.
What are two ways to make an executable Scala program?
There are two general strategies: you can write a script file and use the interpreter, or you can compile an object that has a main method.
What does it mean that Scala is compatible with Java?
The standard Scala backend is a Java Virtual Machine. Scala classes are Java classes and vice versa. You can call the methods of either language from methods in the other one. You can extend Java classes in Scala, and vice versa. The main limitation is that some Scala features do not have equivalents in Java, for example, traits.
What is the latest version of Scala?
This is a simple question to check whether you keep yourself up-to-date with the latest information about Scala or not. If you have not been using Scala actively and don't know the answer then you can say that you have used certain Scala version. Though, the current version of Scala is Scala 2.12, which also require Java 8. Older Scala versions are compatible with Java 6 and above.
Who is the author of Scala Programming language?
This is another basic question about Scala to check your general knowledge about Scala. It is designed by Martin Oderskey, a German computer scientist. He also teaches a course, Functional Programming Principles in Scala and Functional Program Design in Scala on Coursera.
What is the difference between val and var in Scala?
The val keyword stands for value and var stands for variable. You can use keyword val to store values, these are immutable, and cannot change once assigned. On the other hand, keyword var is used to create variables, which are values that can change after being set. If you try to modify a val, the compiler will throw an error. It is similar to the final variable in Java or const in C++.
What is the difference between == in Java and Scala?
Scala has more intuitive notion of equality. The == operator will automatically run the instance's equals method, rather than doing Java style comparison to check that two objects are actually the same reference. By the way, you can still check for referential equality by using eq method. In short, Java == operator compare references while Scala calls the equals() method. You can also read the difference between == and equals() in Java to learn more about how they behave in Java, here.
How to you create Singleton classes in Scala?
Scala introduces a new object keyword, which is used to represent Singleton classes. These are the class with just one instance and their method can be thought of as similar to Java's static methods. Here is a Singleton class in Scala:package test
object Singleton{
def sum(l: List[Int]): Int = l.sum
}
This sum method is available globally, and can be referred to, or imported, as the test.Singleton.sum. A singleton object in Scala can also extend classes and traits.
Which books you have read on Scala so far?
This is a general question to check how did you learn Scala, did you read book or documentation or tutorial etc. It's best to name the book you have read on Scala e.g. I have read Programming in Scala by Martin Odersky and Functional Programming in Scala 1st Edition by Paul Chiusano. If you have not read any book then just say you have not read any book yet and like to read Scala documentation or planning to read a book etc. The most important thing is, to be honest, don't name a book if you have not read it yet. On a different note, try reading a good book on Scala, Martin Odersky's Programming in Scala: Updated for Scala 2.12 is a good starting point.
Which keyword is used to define a function in Scala?
A function is defined in Scala using the def keyword. This may sound familiar to Python developers as Python also uses def to define a function.
What is the use of App class in Scala?
Scala provides a helper class, called App, that provides the main method. Instead of writing your own main method, classes can extend App class to produce concise and executable applications in Scala as shown in the following example:object Main extends App {
Console.println("Hello Scala: " + (args aString ", "))
}
Here, object Main inherits the main method of App trait and args returns the current command line arguments as an array, similar to String[] args in Java main method.
What's the difference between the following terms and types in Scala: 'Nil', 'Null', 'None', and 'Nothing' in Scala?
Answer: Even though they look similar, there are some subtle differences between them, let's see them one by one:
- Nil represents the end of a List.
- Null denotes the absence of value but in Scala, more precisely, Null is a type that represents the absence of type information for complex types that are inherited from AnyRef. It is different than null in Java.
- None is the value of an Option if it has no value in it.
- Nothing is the bottom type of the entire Scala type system, incorporating all types under AnyVal and AnyRef. Nothing is commonly used as a return type from a method that does not terminate normally and throws an exception
What is the difference between an object and a class in Scala?
An object is a singleton instance of a class. It does not need to be instantiated by the developer. If an object has the same name that a class, the object is called a companion object.
What is the difference between a trait and an abstract class in Scala?
Here are some key differences between a trait and an abstract class in Scala:
- A class can inherit from multiple traits but only one abstract class.
- Abstract classes can have constructor parameters as well as type parameters. Traits can have only type parameters. For example, you can’t say trait t(i: Int) {}; the i parameter is illegal.
- Abstract classes are fully interoperable with Java. You can call them from Java code without any wrappers. On the other hand, Traits are fully interoperable only if they do not contain any implementation code. See here to learn more about Abstract class in Java and OOP.
What is the difference between a call-by-value and call-by-name parameter?
The main difference between a call-by-value and a call-by-name parameter is that the former is computed before calling the function, and the later is evaluated when accessed.
What is the case class in Scala?
A case class is a special class definition, which works just like a regular Scala class but compiler creates equals() and toString() methods, as well as immutable access to the field of the class. You can construct case class instance without using the new keyword. One more benefit of the case classes is that instances can be decomposed into their constructed fields and can be used in pattern matching. Here is an example of case class hierarchy in Scala, which consists of an abstract super class Vehicle and two concrete case classes Car and Bikeabstract class Vehicle
case class Car(brand: String) extends Vehicle
case class Bike(brand: String, price: long) extends Vehicle
Instantiating a case class is easy because you don’t need to use the new keyword
val myCar = Car("BMW")
The constructor parameters of case classes are treated as public values and can be accessed directly e.g. myCar.brand will give you "BMW".
Here is another example of traits in Scala using case classes:
What is the difference between a Java method and a Scala function?
Scala function can be treated as a value. It can be assigned to a val or var, or even returned from another function, which is not possible in Java. Though Java 8 brings lambda expression which also makes function as a first class object, which means you can pass a function to a method just like you pass an object as an argument. See here to learn more about the difference between Scala and Java.What is the difference between a Java future and a Scala future?
Even though both Java and Scala's Future object provides asynchronous computation, but there is a subtle difference between them on how you retrieve the result of the computation. Java's Future (java.util.concurrent.Future) requires that you access the result via a blocking get method.Although you can call isDone() method to find out if a Java Future has completed before calling get, thereby avoiding any blocking, you must wait until the Java Future has completed before proceeding with any computation that uses the result. With scala.concurrent.Future you can attach callbacks for completion (success/failure) or simply map it and chain multiple Futures together in a monadic fashion without blocking.
If an object has the same name that a class, the object is called a companion object. A companion object has access to methods of private visibility of the class, and the class also has access to private methods of the object. Doing the comparison with Java, companion objects hold the "static methods" of a class. Just remember that the companion object has to be defined in the same source file that the class. You should also know that Scala classes cannot have static variables or methods. We commonly use Companion Objects for Factories.
What is tail recursion in Scala? What is the benefit?
A function is called tail recursive if the recursive call is the last operation in the function; the method does not rely on the result of the recursive call for any more computation. The most important benefit of the tail recursive function is that compiler can rewrite the method in an iterative fashion, similar to a while loop with mutable state. This allows long, deep recursion without taking too much of stack memory that could result in StackOverFlowError. Unfortunately, Java compiler doesn't support tail call optimization but Scala compiler does. In Scala, you can annotate a function with @tailrec to inform the compiler that this particular method is tail recursive. If the compiler cannot make the function tail recursive, it will throw an error.
What is "traits" in Scala?
Traits are basically Scala's workaround for the JVM not supporting multiple class inheritance. Here is an example of traits in Scala:trait Car {
val brand: String
}
trait Costly{
val cost: Int
}
trait Milege{
val milege: Int
}
class Audi extends Car with Shiny with Miles{
val brand = "Audi"
val cost = 140000
val milege = 20
}
What is Akka, Play, and Sleek in Scala?
Akka is a concurrency framework in Scala which uses Actor based model for building highly concurrent, distributed, and resilient message-driven applications on the JVM. It uses high-level abstractions like Actor, Future, and Stream to simplify coding for concurrent applications. It also provides load balancing, routing, partitioning, and adaptive cluster management. If you are interested in learning Akka, I suggest reading Akka in Action and Akka documentation, both are great resources to learn Akka.
Play is another Scala framework to build web applications in both Java and Scala. The PlayFramework is based on a lightweight, stateless, web-friendly architecture. It is built on Akka, Play provides predictable and minimal resource consumption (CPU, memory, threads) for highly-scalable applications.
Slick is a modern database query and access library for Scala. It allows you to work with stored data almost as if you were using Scala collections while at the same time giving you full control over when a database access happens and which data is transferred. You can write your database queries in Scala instead of SQL, thus profiting from the static checking, compile-time safety, and compositionality of Scala. Slick features an extensible query compiler which can generate code for different backends.
What is Spark?
Apache Spark is the big data and cloud computing framework written in Scala. The Spark has been engineered from the bottom-up for performance, Spark can be 100x faster than Hadoop for large scale data processing by exploiting in memory computing and other optimizations. Spark is also fast when data is stored on disk, and currently, holds the world record for large-scale on-disk sorting. Many developers are now learning Scala for Spark to get into lucrative Big Data Job Market. I recommend reading "Advanced Analytics with Spark: Patterns for Learning from Data at Scale" by Sandy Ryza, Uri Laserson, Sean Owen, and Josh Wills. This book will also teach you how you can use functional programming techniques to solve real-world problems, which is a great skill to have.
What is 'Option' and how is it used in Scala?
The 'Option' in Scala is similar to Optional of Java 8. It is a wrapper type that avoids the occurrence of a NullPointerException in your code by giving you default value in case object is null. When you call get() from Option it can return a default value if the value is null. More importantly, Option provides the ability to differentiate within the type system those values that can be nulled and those that cannot be nulled.
What is ‘Unit’ and ‘()’ in Scala?
The 'Unit' is a type similar to void in Java. You can say it is a Scala equivalent of the void in Java, while still providing the language with an abstraction over the Java platform. The empty tuple '()' is a term representing a Unit value in Scala.
How to compile and run a Scala program?
You can use Scala compiler scalac to compile Scala program (similar to javac) and scala command to run them (similar to scala)
How to tell Scala to look into a class file for some Java class?
Similar to Java, you can use -classpath argument to include a JAR in Scala's classpath, as shown below$ scala -classpath jar
Alternatively, you can also use CLASSPATH environment variable.
What is the difference between a normal class and a case class in Scala?
Following are some key differences between a case class and a normal class in Scala:
- case class allows pattern matching on it.
- you can create instances of case class without using the new keyword
- equals(), hashcode() and toString() method are automatically generated for case classes in Scala
- Scala automatically generate accessor methods for all constructor argument
What is the difference in between Scala and Java package?
Unlike Java, Scala also supports relative package names.What is the difference between 'concurrency' and 'parallelism'? Name some constructs you can use in Scala to leverage both.
Concurrency is when several computations are executing sequentially during overlapping time periods, while parallelism describes processes that are executed simultaneously. The concepts are often confused because actors can be concurrent and parallel, sometimes simultaneously. For example, Node.js has concurrency via its event loop despite being a single threaded implementation. Parallel collections are a canonical example of parallelism, but Futures and the Async library can be as well. In short, Concurrency is about avoiding access to mutable state by multiple threads at the same time. Parallelism is taking a single task and breaking it apart to be performed by multiple threads at the same time.
What are two ways to make an executable Scala program?
There are two general strategies: you can write a script file and use the interpreter, or you can compile an object that has a main method.
What does it mean that Scala is compatible with Java?
The standard Scala backend is a Java Virtual Machine. Scala classes are Java classes and vice versa. You can call the methods of either language from methods in the other one. You can extend Java classes in Scala, and vice versa. The main limitation is that some Scala features do not have equivalents in Java, for example, traits.Scala Functional Programming Interview Questions
What is Monad in Scala?
A monad is an object that wraps another object in Scala. It helps to perform the data manipulation of the underlying object, instead of manipulating the object directly.
What are High Order Functions in Scala?
High order functions are functions that can receive or return other functions. Common examples in Scala are the filter, map, and flatMap functions, which receive other functions as arguments.
What is a Closure in Scala?
A closure is also known as an anonymous function whose return value depends upon the value of the variables declared outside the function.
What is currying in Scala?
Currying is a technique to transform a function that takes multiple arguments into a function that takes a single argument. There is some feature which is inspired by other function programming languages like Haskell and lisp. If you want to learn more about functional programming techniques in Scala, I suggest you reading Paul Chiusano and Rúnar Bjarnason's Functional Programming in Scala 1st edition. It is one of the best books to learn Functional Programming regardless of language. This book teaches you both Scala and Functional Programming through examples and exercise where you use key features of Scala by yourself.
4) What is the advantage of Scala?
a) Less error prone functional style
b) High maintainability and productivity
c) High scalability
d) High testability
e) Provides features of concurrent programming
5) In what ways Scala is better than other programming language?
a) The arrays uses regular generics, while in other language, generics are bolted on as an afterthought and are completely separate but have overlapping behaviours with arrays.
b) Scala has immutable “val” as a first class language feature. The “val” of scala is similar to Java final variables. Contents may mutate but top reference is immutable.
c) Scala lets ‘if blocks’, ‘for-yield loops’, and ‘code’ in braces to return a value. It is more preferable, and eliminates the need for a separate ternary operator.
d) Singleton has singleton objects rather than C++/Java/ C# classic static. It is a cleaner solution
e) Persistent immutable collections are the default and built into the standard library.
f) It has native tuples and a concise code
g) It has no boiler plate code
6) What are the Scala variables?
Values and variables are two shapes that come in Scala. A value variable is constant and cannot be changed once assigned. It is immutable, while a regular variable, on the other hand, is mutable, and you can change the value.
The two types of variables are
var myVar : Int=0;
val myVal: Int=1;
7) Mention the difference between an object and a class ?
A class is a definition for a description. It defines a type in terms of methods and composition of other types. A class is a blueprint of the object. While, an object is a singleton, an instance of a class which is unique. An anonymous class is created for every object in the code, it inherits from whatever classes you declared object to implement.
8) What is recursion tail in scala?
‘Recursion’ is a function that calls itself. A function that calls itself, for example, a function ‘A’ calls function ‘B’, which calls the function ‘C’. It is a technique used frequently in functional programming. In order for a tail recursive, the call back to the function must be the last function to be performed.
9) What is ‘scala trait’ in scala?
‘Traits’ are used to define object types specified by the signature of the supported methods. Scala allows to be partially implemented but traits may not have constructor parameters. A trait consists of method and field definition, by mixing them into classes it can be reused.
10) When can you use traits?
There is no specific rule when you can use traits, but there is a guideline which you can consider.
a) If the behaviour will not be reused, then make it a concrete class. Anyhow it is not a reusable behaviour.
b) In order to inherit from it in Java code, an abstract class can be used.
c) If efficiency is a priority then lean towards using a class
d) Make it a trait if it might be reused in multiple and unrelated classes. In different parts of the class hierarchy only traits can be mixed into different parts.
e) You can use abstract class, if you want to distribute it in compiled form and expects outside groups to write classes inheriting from it.
11) What is Case Classes?
Case classes provides a recursive decomposition mechanism via pattern matching, it is a regular classes which export their constructor parameter. The constructor parameters of case classes can be accessed directly and are treated as public values.
12) What is the use of tuples in scala?
Scala tuples combine a fixed number of items together so that they can be passed around as whole. A tuple is immutable and can hold objects with different types, unlike an array or list.
13) What is function currying in Scala?
Currying is the technique of transforming a function that takes multiple arguments into a function that takes a single argument Many of the same techniques as language like Haskell and LISP are supported by Scala. Function currying is one of the least used and misunderstood one.
14) What are implicit parameters in Scala?
Implicit parameter is the way that allows parameters of a method to be “found”. It is similar to default parameters, but it has a different mechanism for finding the “default” value. The implicit parameter is a parameter to method or constructor that is marked as implicit. This means if a parameter value is not mentioned then the compiler will search for an “implicit” value defined within a scope.
15) What is a closure in Scala?
A closure is a function whose return value depends on the value of the variables declared outside the function.
16) What is Monad in Scala?
A monad is an object that wraps another object. You pass the Monad mini-programs, i.e functions, to perform the data manipulation of the underlying object, instead of manipulating the object directly. Monad chooses how to apply the program to the underlying object.
17) What is Scala anonymous function?
In a source code, anonymous functions are called ‘function literals’ and at run time, function literals are instantiated into objects called function values. Scala provides a relatively easy syntax for defining anonymous functions.
18) Explain ‘Scala higher order’ functions?
Scala allows the definition of higher order functions. These are functions that take other functions as parameters, or whose result is a function. In the following example, apply () function takes another function ‘f’ and a value ‘v’ and applies function to v.
Example:
When the above code is compiled and executed, it produces following result.
19) What is the difference between var and value?
In scala, you can define a variable using either a, val or var keywords. The difference between val and var is, var is much like java declaration, but val is little different. We cannot change the reference to point to another reference, once the variable is declared using val. The variable defined using var keywords are mutable and can be changed any number of times.
20) What are option, some and none in scala?
‘Option’ is a Scala generic type that can either be ‘some’ generic value or none. ‘Queue’ often uses it to represent primitives that may be null.
21) How do I append to the list?
In scala to append into a list, use “:+” single value
22) How can you format a string?
To format a string, use the .format () method, in scala you can use
Val formatted= “%s %i”.format (mystring.myInt)
23) Why scala prefers immutability?
Scala prefers immutability in design and in many cases uses it as default. Immutability can help when dealing with equality issues or concurrent programs.
24) What are the four types of scala identifiers ?
The four types of identifiers are
a) Alpha numeric identifiers
b) Operator identifiers
c) Mixed identifiers
d) Literal identifiers
25) What are the different types of Scala literals?
The different types of literals in scala are
a) Integer literals
b) Floating point literals
c) Boolean literals
d) Symbol literals
e) Character literals
f) String literals
g) Multi-Line strings
What is Monad in Scala?
A monad is an object that wraps another object in Scala. It helps to perform the data manipulation of the underlying object, instead of manipulating the object directly.What are High Order Functions in Scala?
High order functions are functions that can receive or return other functions. Common examples in Scala are the filter, map, and flatMap functions, which receive other functions as arguments.
What is a Closure in Scala?
A closure is also known as an anonymous function whose return value depends upon the value of the variables declared outside the function.
What is currying in Scala?
Currying is a technique to transform a function that takes multiple arguments into a function that takes a single argument. There is some feature which is inspired by other function programming languages like Haskell and lisp. If you want to learn more about functional programming techniques in Scala, I suggest you reading Paul Chiusano and Rúnar Bjarnason's Functional Programming in Scala 1st edition. It is one of the best books to learn Functional Programming regardless of language. This book teaches you both Scala and Functional Programming through examples and exercise where you use key features of Scala by yourself.
4) What is the advantage of Scala?
a) Less error prone functional style
b) High maintainability and productivity
c) High scalability
d) High testability
e) Provides features of concurrent programming
5) In what ways Scala is better than other programming language?
a) The arrays uses regular generics, while in other language, generics are bolted on as an afterthought and are completely separate but have overlapping behaviours with arrays.
b) Scala has immutable “val” as a first class language feature. The “val” of scala is similar to Java final variables. Contents may mutate but top reference is immutable.
c) Scala lets ‘if blocks’, ‘for-yield loops’, and ‘code’ in braces to return a value. It is more preferable, and eliminates the need for a separate ternary operator.
d) Singleton has singleton objects rather than C++/Java/ C# classic static. It is a cleaner solution
e) Persistent immutable collections are the default and built into the standard library.
f) It has native tuples and a concise code
g) It has no boiler plate code
6) What are the Scala variables?
Values and variables are two shapes that come in Scala. A value variable is constant and cannot be changed once assigned. It is immutable, while a regular variable, on the other hand, is mutable, and you can change the value.
The two types of variables are
var myVar : Int=0;
val myVal: Int=1;
7) Mention the difference between an object and a class ?
A class is a definition for a description. It defines a type in terms of methods and composition of other types. A class is a blueprint of the object. While, an object is a singleton, an instance of a class which is unique. An anonymous class is created for every object in the code, it inherits from whatever classes you declared object to implement.
8) What is recursion tail in scala?
‘Recursion’ is a function that calls itself. A function that calls itself, for example, a function ‘A’ calls function ‘B’, which calls the function ‘C’. It is a technique used frequently in functional programming. In order for a tail recursive, the call back to the function must be the last function to be performed.
9) What is ‘scala trait’ in scala?
‘Traits’ are used to define object types specified by the signature of the supported methods. Scala allows to be partially implemented but traits may not have constructor parameters. A trait consists of method and field definition, by mixing them into classes it can be reused.
10) When can you use traits?
There is no specific rule when you can use traits, but there is a guideline which you can consider.
a) If the behaviour will not be reused, then make it a concrete class. Anyhow it is not a reusable behaviour.
b) In order to inherit from it in Java code, an abstract class can be used.
c) If efficiency is a priority then lean towards using a class
d) Make it a trait if it might be reused in multiple and unrelated classes. In different parts of the class hierarchy only traits can be mixed into different parts.
e) You can use abstract class, if you want to distribute it in compiled form and expects outside groups to write classes inheriting from it.
11) What is Case Classes?
Case classes provides a recursive decomposition mechanism via pattern matching, it is a regular classes which export their constructor parameter. The constructor parameters of case classes can be accessed directly and are treated as public values.
12) What is the use of tuples in scala?
Scala tuples combine a fixed number of items together so that they can be passed around as whole. A tuple is immutable and can hold objects with different types, unlike an array or list.
13) What is function currying in Scala?
Currying is the technique of transforming a function that takes multiple arguments into a function that takes a single argument Many of the same techniques as language like Haskell and LISP are supported by Scala. Function currying is one of the least used and misunderstood one.
14) What are implicit parameters in Scala?
Implicit parameter is the way that allows parameters of a method to be “found”. It is similar to default parameters, but it has a different mechanism for finding the “default” value. The implicit parameter is a parameter to method or constructor that is marked as implicit. This means if a parameter value is not mentioned then the compiler will search for an “implicit” value defined within a scope.
15) What is a closure in Scala?
A closure is a function whose return value depends on the value of the variables declared outside the function.
16) What is Monad in Scala?
A monad is an object that wraps another object. You pass the Monad mini-programs, i.e functions, to perform the data manipulation of the underlying object, instead of manipulating the object directly. Monad chooses how to apply the program to the underlying object.
17) What is Scala anonymous function?
In a source code, anonymous functions are called ‘function literals’ and at run time, function literals are instantiated into objects called function values. Scala provides a relatively easy syntax for defining anonymous functions.
18) Explain ‘Scala higher order’ functions?
Scala allows the definition of higher order functions. These are functions that take other functions as parameters, or whose result is a function. In the following example, apply () function takes another function ‘f’ and a value ‘v’ and applies function to v.
Example:
When the above code is compiled and executed, it produces following result.
19) What is the difference between var and value?
In scala, you can define a variable using either a, val or var keywords. The difference between val and var is, var is much like java declaration, but val is little different. We cannot change the reference to point to another reference, once the variable is declared using val. The variable defined using var keywords are mutable and can be changed any number of times.
20) What are option, some and none in scala?
‘Option’ is a Scala generic type that can either be ‘some’ generic value or none. ‘Queue’ often uses it to represent primitives that may be null.
21) How do I append to the list?
In scala to append into a list, use “
:+” single value
22) How can you format a string?
To format a string, use the .format () method, in scala you can use
Val formatted= “%s %i”.format (mystring.myInt)
23) Why scala prefers immutability?
Scala prefers immutability in design and in many cases uses it as default. Immutability can help when dealing with equality issues or concurrent programs.
24) What are the four types of scala identifiers ?
The four types of identifiers are
a) Alpha numeric identifiers
b) Operator identifiers
c) Mixed identifiers
d) Literal identifiers
25) What are the different types of Scala literals?
The different types of literals in scala are
a) Integer literals
b) Floating point literals
c) Boolean literals
d) Symbol literals
e) Character literals
f) String literals
g) Multi-Line strings
Comments
Post a Comment